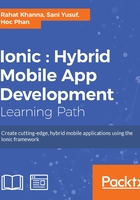
Ionic JS components
CSS is used for styling mobile components but JavaScript is used to write logic and create the user experience in a Hybrid App. Ionic has reusable Angular directives that help developers create smooth mobile-specific experiences. Apart from directives, Ionic also has controllers and services in its library to help create mobile-specific components. We will be discussing the most important components in this section of the chapter.
Actionsheet – $ionicActionSheet
The actionsheet
component is a sliding panel that comes from the bottom and can contain multiple buttons intended to perform some actions in the Mobile App. This component has been inspired from the actionsheet
component in iOS. $ionicActionSheet
is a service in Ionic Library that can be injected in any controller and initiated using the show
method.
The show
method inputs a set of options mentioned in the following table to control the actionsheet
component.

The code for an example is as follows:
// Action Sheet Initialization using Properties var sheetId = $ionicActionSheet.show({ titleText: 'Manage Books', buttons: [ { text: '<b>Add New Book </b>' } ], cancelText: 'Cancel', destructiveText: 'Delete Book', cancel: function() { // code when cancel button is clicked }, buttonClicked: function(index) { // code which is to be executed on button click } });
Backdrop - $ionicBackdrop
A backdrop is a transparent blackish UI screen that appears behind popups, loading, and other overlays. Multiple UI components require a backdrop, but only one backdrop appears in DOM. In order to use a backdrop, any component can call the retain
method, and whenever its usage is over the component can call the release
method.
The code for demonstrating its usage is as follows:
$stateProvider.state('homeView',{ //Show a backdrop for one second $scope.action = function() { $ionicBackdrop.retain(); $timeout(function() { $ionicBackdrop.release(); }, 1000); }; });
Form inputs
Apart from the normal input elements to take text format inputs, there are special form input directives for checkboxes, radio, and toggle. We have already discussed the CSS styles used for the same, but you can use directives themselves to achieve the functionality along with the style.
This directive adds the required styles to a checkbox. It renders like a normal angular checkbox:
ion-checkbox ng-model="isChecked">Checkbox Label</ion-checkbox>
The <
ion-radio>
directive is also used to style the radio input according to the mobile UI. In order to create a set of radio buttons, please give the same ng-model
property to all and use ng-value
to assign a value for a specific radio element:
<ion-radio ng-model="option" ng-value="'1'">Option 1</ion-radio> <ion-radio ng-model="option" ng-value="'2'">Option 2</ion-radio>
Gestures and events
In a Mobile App, gestures and events are very important in shaping the user experience. The event handling should be perfect and there should be a multitude of events available to developers for adding appropriate actions for different events. In Ionic Framework, there are different event directives that can be used to bind callbacks for those events. Also, there is an $ionicGesture
service that can be used to programmatically bind the events with callbacks.
The $ionicGesture service
The methods available under the $ionicGesture
service are given as follows.
Gesture events
A list of gesture events available in Ionic Framework are given in the following table:

Lists
List is the most popular component used in any Mobile App. Generally, all the data displayed in a mobile view is in the form of a list. A list can have multiple list items representing rows. A list item can contain any content ranging from text to icons and custom elements.
This directive represents a list in Ionic Framework. This directive supports complex interactions such as drag to reorder, swipe to edit, and removing items. The <ion-list>
directive can consist of one or more <ion-item>
directives representing each row. There are other directives augmenting its functionality such as <ion-option-button>
used for swipe to edit buttons, <ion-reorder-button>
shown while reorder is enabled, and <ion-delete-button>
shown while deleting a row item.
The attributes available for this directive are given in the following table:

The following code explains the usage :
// Example with complex Scenario <ion-list ng-controller="AppCtrl" show-delete="shouldShowDelete" show-reorder="shouldShowReorder" can-swipe="listCanSwipe"> <ion-item ng-repeat="row in rows" class="item-thumbnail-left"> <h2>{{item.title}}</h2> <p>{{item.description}}</p> <ion-option-button class="button-positive" ng-click="share(item)"> Share </ion-option-button> <ion-option-button class="button-info" ng-click="edit(item)"> Edit </ion-option-button> <ion-delete-button class="ion-minus-circled" ng-click="items.splice($index, 1)"> </ion-delete-button> <ion-reorder-button class="ion-navicon" on-reorder="reorderItem(item, $fromIndex, $toIndex)"> </ion-reorder-button> </ion-item> </ion-list>
Loading – $ionicLoading
$ionicLoading
is an angular service that controls the display of an overlay representing a loader. The loading indicator can be configured by passing certain options to the show
method of this service.
The following is the usage of this service:
// Sample Module angular.module('MyApp', ['ionic']) controller('MyCtrl', function($scope, $ionicLoading) { $scope.show = function() { $ionicLoading.show({ template: 'Loading...' }); }; $scope.hide = function(){ $ionicLoading.hide(); }; });
The important options available to be passed to the show method are:

Modal – $ionicModal
This is a service that controls displaying a popup over the existing UI temporarily. It uses the <ion-modal-view>
directive in its template to represent the modal DOM element. It is used for generally editing an item or showing a dialog box or a choice box. There are two methods available on the $ionicModal
service, fromTemplate
and fromTemplateUrl
, which takes input as template string
or template url
respectively as a first parameter. The second argument to both methods is the options
object, which is passed to the initialize method of the IonicModal
controller instance.
This is a controller instantiated by the $ionicModal
service. The ionicModal
method remove
should be called after the use of the modal is over in order to avoid memory leaks.
The different methods available to this controller are as follows.
It creates a new modal controller instance. It takes as an argument an options
object, which should contain the following properties:
scope (object)
: The scope to be a child of.animation (string)
: The animation to show and hide. The default isslide-in-up
.focusFirstInput (boolean)
: The control whether to focus first input in modal or not.backdropClickToClose (boolean)
: Whether to close modal on clicking backdrop.hardwareBackButtonClose (boolean)
: The control to decide whether modal should be closed using hardware back button or not.show()
: This method is used to show the modal instance.hide()
: This method is used to hide the modal instance.remove()
: This method is used to remove the modal instance from memory.isShown()
: This method returns a boolean representing whether the modal is shown or not.
Popover – $ionicPopover
A Popover component is also modeled similarly to modal and has two objects, the $ionicPopover
service and the ionicPopover
controller. Popover is a view that floats above the app's content. Popover can be used to display extra information or take input for a choice. The content of a Popover needs to be put inside an <ion-popover-view>
element.
The $ionicPopover
service exposes similar methods such as the $ionicModal
service—fromTemplate
and fromTemplateUrl
. The ionicPopover
controller also has similar events to the ionicModal
controller. The initialize
method for this controller has the options
object as input but it does not contain the property animation
.
Spinner – ion-spinner
In a mobile UI or views, only a particular section of view needs to be refreshed or loaded and a spinner/loader needs to be displayed in that section. <ion-spinner>
is a directive that displays loader icons. There are multiple available icons such as spiral, ripple, dots, lines, and so on. The theme colors for Ionic can also be used along with using class names such as spinner-<theme>
.
The following is the usage of this directive:
<ion-spinner icon="spiral spinner-calm"></ion-spinner>