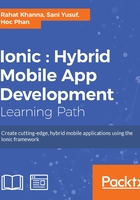
The Ionic starter template
In the Ionic CLI, you can start a new project using a specific template. There are predefined templates available in the Ionic CLI toolset. They all provide a starting point for developers and also act as a skeleton for your new app. We have already seen the command to start a new project with a specific template earlier on in this chapter. If we want to choose the tabs
template, then the command would look like the following:
$ ionic start TabsDemo tabs
We will be discussing the important files in each of the templates.
The blank template
It contains the bare minimum code for an Ionic App. It injects Ionic and Angular dependencies into your index.html
and creates separate folders for CSS, js
, and img
. It also provides a basic view code in the body tag of index.html
to show ion-pane
and ion-header
content.
The tabs template
The tabs
template provides an app layout with the bottom three tabs and multiple views for each tab. It sets up the controllers and templates for different states. In Ionic, the open source and popular router called UI-Router is used for creating states/routes. We will learn about it in detail in the next chapter.
In the app.js
config
block, the states are defined using the $stateProvider
object. In this template, an abstract state is created for the tabs as it will be shown for all views and then the states for subsequent views are created.
In index.html
, the <ion-nav-bar>
directive is used to display a top navbar on all the views. It also contains the <ion-nav-view>
directive that will act as a placeholder for all the default layout/templates associated with the abstract state for showing the tabs on all views of the app:
<ion-nav-bar class="bar-stable"> <ion-nav-back-button> </ion-nav-back-button> </ion-nav-bar> <ion-nav-view></ion-nav-view>
All the template files are present in the templates
folder inside the www
folder. The tabs.html
file is associated with the main tabs state and uses the <ion-tabs>
directive, which consists of one or more <ion-tab>
directives to represent each tab. We can set specific properties of the directive such as icon-on
and icon-off
to show an active icon and non-active icon respectively. We also create named <ion-nav-view>
directives under each tab directive to create containers for showing templates for different tabs:
<ion-tabs class="tabs-icon-top tabs-color-active-positive"> <!-- Dashboard Tab --> <ion-tab title="Status" icon-off="ion-ios-pulse" icon-on="ion-ios-pulse-strong" href="#/tab/dash"> <ion-nav-view name="tab-dash"></ion-nav-view> </ion-tab> <!-- Chats Tab --> <ion-tab title="Chats" icon-off="ion-ios-chatboxes-outline" icon-on="ion-ios-chatboxes" href="#/tab/chats"> <ion-nav-view name="tab-chats"></ion-nav-view> </ion-tab> <!-- Account Tab --> <ion-tab title="Account" icon-off="ion-ios-gear-outline" icon-on="ion-ios-gear" href="#/tab/account"> <ion-nav-view name="tab-account"></ion-nav-view> </ion-tab> </ion-tabs>
The templates
folder also has a separate file for each tab view named tab-<view_name>.html
. The code in each file consists of one <ion-view>
directive on the top with the view-title
attribute to set the header bar for our app. It also consists of an <ion-content>
directive to contain the actual content of that particular view.
The controllers for each of the views are bound in the app.js
in the respective state representing the tab view for account, chats, and dashboard.
The sidemenu template
The sidemenu
template contains a layout where a side menu drawer is used for primary navigation. Ionic Project can be created with this template by mentioning the name of this template in the Ionic start command as follows:
$ ionic start MenuDemo sidemenu
This template has only the <ion-nav-view>
directive in the body
tag of index.html
. The main state that is marked as abstract in app.js
will load the template templates/menu.html
and associate the controller AppCtrl
with it. According to the other states defined in app.js
, respective templates will be loaded into the menucontent
ion-view
directive present in menu.html
. The code for menu.html
should be as follows:
<ion-side-menus enable-menu-with-back-views="false"> <ion-side-menu-content> <ion-nav-bar class="bar-stable"> <ion-nav-back-button> </ion-nav-back-button> <ion-nav-buttons side="left"> <button class="button button-icon button-clear ion-navicon" menu-toggle="left"> </button> </ion-nav-buttons> </ion-nav-bar> <ion-nav-view name="menuContent"></ion-nav-view> </ion-side-menu-content> <ion-side-menu side="left"> <ion-header-bar class="bar-stable"> <h1 class="title">Left</h1> </ion-header-bar> <ion-content> <ion-list> <ion-item menu-close ng-click="login()"> Login </ion-item> <ion-item menu-close href="#/app/search"> Search </ion-item> <ion-item menu-close href="#/app/browse"> Browse </ion-item> <ion-item menu-close href="#/app/playlists"> Playlists </ion-item> </ion-list> </ion-content> </ion-side-menu> </ion-side-menus>
The ion-content
directive in this file contains an <ion-side-menu-content>
directive, which contains the view displayed in the center or the main view. It contains another subview <ion-nav-view name="menucontent">
, which will show respective templates for each state representing the menu item views. The links to the views are contained in the <ion-content>
directive under <ion-list>
.
The maps template
The maps
template contains a layout where a map is loaded on the main view itself. It includes the controller for the view and a directive for showing the map on Ionic App. It has a feature of showing the current position on the map. In order to create a project using this template, please use the following command:
$ ionic start MapsDemo maps
The index.html
file contains an <ion-header>
directive, an <ion-content>
directive containing the custom <map>
directive, and the <ion-footer>
directive. It is important to load the Google Maps API script to display the map. The controller is associated on the body
tag using the ng-controller
directive attribute:
<body ng-app="starter" ng-controller="MapCtrl"> <ion-header-bar class="bar-stable"> <h1 class="title">Map</h1> </ion-header-bar> <ion-content scroll="false"> <map on-create="mapCreated(map)"></map> </ion-content> <ion-footer-bar class="bar-stable"> <a ng-click="centerOnMe()" class="button button-icon icon ion-navigate"></a> </ion-footer-bar> <script src="https://maps.googleapis.com/maps/api/js?key=AIzaSyB16sGmIekuGIvYOfNoW9T44377IU2d2Es&sensor=true"> </script> </body>