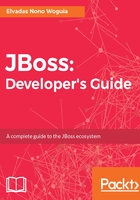
Implementing a clustered money transfer web page
The clustered money transfer app is implemented in the jossdevguidebook/chapters/ch2 subfolder, and two main modules are used to implement the following details:
- Core project beosbank-core: This project hosts the model and domain classes. To keep it simple, we have the following entities in the com.beosbank.jbdevg.jbossas.domain package:
- Entity Customer: A beosBank customer who can act as a sender or receiver in a money transfer operation.
- Entity Address: Customer address, including country names, in order to handle cross-border remittances. A customer has one and only one address.
- Entity MoneyTransfer: This represents a money transfer transaction between one sender and one receiver. The money transfer can have one status listed by the MoneyTransferStatusEnum.
The money transfer entity also has attributes to store various prices, taxes, and currencies to satisfy the transaction.
- Web project beosbank-web/: This project holds the web part of the application. It is a JSF2 application including various API dependencies from JBoss maven repositories. The webapp depends on the beosbank-core artifact. This web app implements one use case: send a money transfer request to a recipient through three tabs/steps. These steps are implemented with the Primefaces Wizard component. The wizard component allows users to navigate steps back and forth:

The Transfer details tab, is used to collect basic information of the money transfer: sender and receiver countries and names, and the amount to send.
The Payment Infos tab is designed to collect and/or select payment methods. For the purpose of this lab, the tab has only one field associated to a credit card number.
The Confirmation tab is a confirmation page where all the information previously entered by the user is displayed for verification before submitting the money transfer request.
Technically, the view is a JSF page implemented in the index.xhtml file. The view is bound to a ManageBean Request Controller named mtvcBean of the MoneyTransferVviewControllerBean type:
@RequestScoped
@ManagedBean(name="mtvcBean")
public class MoneyTransferViewController implements Serializable {
private static final long serialVersionUID = 1L;
private Map<String,String> countries;
private Map<String,String> currencies;
private MoneyTransfer transfer;
@PostConstruct
public void init() {
//Initialize countries and currencies list
initCountries();
initCountriesCurrencies();
//Load Money Transfer data from session if any
getMoneyTransferDataFromSessionOrCreateNew();
}
....
//load the money transfer object from the Session
public MoneyTransfer getMoneyTransferDataFromSessionOrCreateNew() {
HttpSession session = getSession();
transfer = (MoneyTransfer)session.getAttribute("transfer");
if(transfer==null){
transfer=new MoneyTransfer();
session.setAttribute("transfer",this.transfer);
}
return transfer;
}
}
The MoneyTransferVviewControllerBean bean as an attribute of the MoneyTransfer type to collect user inputs. The transfer injection in the bean is customized to first check whether there is a pending transfer object in the HTTP session; if yes, the session object is used.
During the sending process, a simple test to validate the session replication is to kill the running node when a user completes the second step of the wizard. While recovering his session on the failover node, data entered in the first tab (transfer details) should be available.
When was this bean placed in the session? For the first call, the getMoneyTransferDataFromSessionOrCreateNew method will not find any transaction object in the user session; a new object will therefore be created and set as a transaction attribute in the mtvcBean bean.
The mtvcBean bean and the httpSession object have the same reference on transfer objects. Whenever user inputs data, it will update both the transfer object of the mtcvBean and the transfer attribute of the httpSession:
public void setTransfer(MoneyTransfer transfer) {
this.transfer = transfer;
HttpSession session = getSession();
session.setAttribute("transfer",this.transfer);
}
In your application workflow, you can also set up specific synchronization points to set attributes in the session. For this demo, you can look at the onReceiverCountryChange event.
Whenever the sender selects a new value in the drop-down list for the receiver country, the transfer attribute is set on the httpSession object.
Once the application is ready, we need to deploy it on server instances
In the following sections, we will see how to deploy the money transfer web application on server group dev03 (instances node13 and node23), using two techniques.
- JBoss CLI
- JBoss-as maven plugin