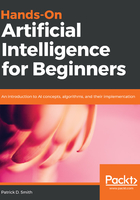
Basic building blocks
As you may have guessed from the name, TensorFlow relies on the algebraic concept of tensors that we learned about in the previous chapter. Everything, from input data to parameters, is stored in a tensor in TensorFlow. As such, TensorFlow has its own functions for many of the basic operations normally handled by NumPy.
When writing tensors in TensorFlow, we're really writing everything in an array structure. Remember how an array can be a rank 1 tensor? That is exactly what we are passing in the preceding example. If we wanted to pass a rank 3 tensor, we'd simply write x = tf.constant([1,2,3,4],[5,6,7,8],[9,10,11,12]). You'll notice that we defined constants in the following code; these are just one of three types of data structure we can use in TensorFlow:
- Constants: Defined values that cannot change
- Placeholders: Objects that will be assigned a value during a TensorFlow session
- Variables: Like constants, only the values can change
Alright, back to the code. If we had run the following code block, we would have been left with a TensorFlow object, which looks something like tensor ("Mul:0", shape=(4,), dtype=int32).
## Import Tensorflow at tf for simplicity
import tensorflow as tf
## Define two constants
const1 = tf.constant([4])
const2 = tf.constant([5])
## Multiply the constants
product = tf.multiply(const1, const2)
Why? because TensorFlow runs on the concept of sessions. The underlying code of TensorFlow is written in C++, and a session allows a high-level TensorFlow package to communicate with the low-level C++ runtime.
## Variable Initializer
init = initialize_all_variables()
## Initialize the session
sess = tf.Session()
sess.run(init)
## Run the session
print(sess.run(product))
## Close the session
sess.close()
One last important concept in TensorFlow is that of scopes. Scopes help us control various operational blocks within our model:
with tf.name_scope("my_scope"):
## Define two constants
const1 = tf.constant([4])
const2 = tf.constant([5])
## Multiply the constants
product = tf.multiply(const1, const2)
That's it! We've successfully performed out first operation in TensorFlow. We'll also be learning more about the in-depth operations of TensorFlow in the next chapter on building Artificial Neural Networks (ANNs).