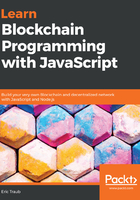
Blockchain constructor function
Let's get started with building our blockchain data structure. We'll start by opening all of the files that we have in our blockchain directory by using the Sublime editor. If you are comfortable using any other editor, you can use that too. Open our entire blockchain directory in whichever editor you prefer.
We'll be building our entire blockchain data structure in the dev/blockchain.js file that we created in Chapter 1, Setting up the Project. Let's build this blockchain data structure by using a constructor function that we learned about in the previous section. So, let's begin:
For the constructor by type the following:
function Blockchain () {
}
For now, the Blockchain () function is not going to take any parameters.
Next, inside of our constructor function, we are going to add the following terms:
function Blockchain () {
this.chain = [];
this.newTransactions = [];
}
In the preceding code block, [] defines an array, and the this.chain = []; is where the meat of our blockchain will be stored. All of the blocks that we mine will be stored in this particular array as a chain, while this.newTransactions = []; is where we will hold all of the new transactions that are created before they are placed into a block.
All of this might seem a little bit confusing and overwhelming right now, but don't worry about it. Let's dive deeper into this in future sections.
When defining the preceding function, we have initiated the process of creating the blockchain data structure. Now, you might be wondering why we are using a constructor function to build our blockchain data structure instead of a class; the answer to this is that this is simply a preference. We prefer to create constructor functions over classes in JavaScript because in JavaScript there really are no classes. Classes in JavaScript are simply a kind of a sugar coating on top of constructor functions and the object prototype. So, we simply prefer to just stick with constructor functions.
However, if you want to create a blockchain by using a class, you could do something such as in the following block of code:
class Blockchain {
constructor() {
this.chain = [];
this.newTransactions = [];
}
// Here you can build out all of the methods
// that we are going to write inside of this
// Blockchain class.
}
So, either way, if you prefer using a constructor function or using a class, it would work just fine.
This is it – with defining our function, we've began the process of building our blockchain data structure. In further sections, we'll continue to build on this.