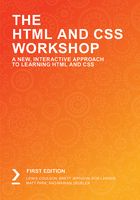
Breadcrumbs
On websites that have lots of pages, it is common for pages to contain what is called breadcrumbs. This is a list of links that easily allow the user to see the context of the current page within the website structure and to easily navigate to the parent pages. The following screenshot shows a breadcrumb taken from the Packt website, https://packt.live/2q0AbGZ:

Figure 3.27: Breadcrumb from the Packt web page
As you can see in the preceding figure, web pages can contain many parent pages or categories. The breadcrumbs of the current page are for a book in the Packt online store. Moving from right to left, the breadcrumb links get more specific, moving from the home page to the closest parent page. The user is free to click on any link, which will take them back to a page related to the current page.
Exercise 3.03: Breadcrumb
In this exercise, we will step through the process of writing the HTML and CSS for the breadcrumb component shown in Figure 3.22. The following figure shows it in more detail:

Figure 3.28: Breadcrumb
The following are the steps to complete this exercise:
- First, let's create a file called breadcrumb.html in VSCode using the following HTML code as a starter file:
<!DOCTYPE html>
<html>
<head>
<title>Exercise 3.03</title>
<style>
/* your CSS will go here */
</style>
</head>
<body>
<!-- your HTML will go here -->
</body>
</html>
- First, we need to decide what the best HTML tag will be for the breadcrumb. Since this is a list of links and the ordering is important, we will use ol. We will place this in between the body tags as follows:
<body>
<ol class="breadcrumb">
<li>Home</li>
<li>Used DVDs</li>
<li>Less than £10</li>
</ol>
</body>
- We will then add anchors to all but the last item because the last item represents the current page the user is viewing so doesn't need to be clickable:
<body>
<ol class="breadcrumb">
<li><a href="">Home</a></li>
<li><a href="">Used DVDs</a></li>
<li>Less than £10</li>
</ol>
</body>
- Let's now take a look at what this will look like in the browser:
Figure 3.29: Default breadcrumb
- We will now start adding our styling. Again, we will start with a basic CSS reset and we will add the styles in between the opening and closing style tags as follows:
<style>
* {
margin: 0;
padding: 0;
}
</style>
- We will use the flex layout technique again, which we learned about in the previous chapter, and remove the default numbers from the ordered list:
<style>
* {
margin: 0;
padding: 0;
}
.breadcrumb {
display: flex;
list-style: none;
}
</style>
- Let's style the list items in the ordered list. We will first add some padding to the list items. Then, we will add a forward slash at the end of all the list items except the last one. We will add some margin to the left to ensure our list items are nicely separated:
.breadcrumb li {
padding: 10px;
}
.breadcrumb li:after {
content: '/';
margin-left: 20px;
}
.breadcrumb li:last-child:after {
content: '';
}
- Finally, we will style the anchors, making sure the color of the text is black and only showing an underline when the user hovers over the link:
.breadcrumb a {
color: black;
text-decoration: none;
}
.breadcrumb a:hover {
text-decoration: underline;
}
If you now right-click on the filename in VSCode on the left-hand side of the screen and select open in default browser, you will see the breadcrumb component in your browser.
You should now see something similar to the following figure in your browser:

Figure 3.30: Styled breadcrumb
Exercise 3.04: Page Heading and Introduction
We will now write the HTML and CSS for the heading and introduction section of the wireframe. The following figure shows it in more detail:

Figure 3.31: Introduction section
The steps to complete the exercise are as follows:
- First, let's create a file called text.html in VSCode using the following HTML code as a starter file:
<!DOCTYPE html>
<html>
<head>
<title>Exercise 3.04</title>
<style>
/* your CSS will go here */
</style>
</head>
<body>
<!-- your HTML will go here -->
</body>
</html>
- First, let's decide on the correct markup to use. Since the heading represents the top-level heading for the page, we will use h1 and we will use a plain p for the introduction. We will place the HTML in between the opening and closing body tags:
<body>
<section class="intro">
<h1>Videos less than £10</h1>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. In bibendum non purus quis vestibulum. Pellentesque ultricies quam lacus, ut tristique sapien tristique et.</p>
</section>
</body>
- Let's now take a look at what this looks like in the browser:
Figure 3.32: Default introduction section
- This is actually pretty close to how we want these elements to look. We will just adjust the heading margin and the line height for the paragraph to make the text more readable. We will place the CSS in between the opening and closing style tags as follows:
<style>
.intro {
margin: 30px 0;
padding-left: 10px;
width: 50%;
}
.intro h1 {
margin-bottom: 15px;
}
.intro p {
line-height: 1.5;
}
</style>
If you now right-click on the filename in VSCode on the left-hand side of the screen and select open in default browser, you will see the heading and paragraph.
You should now see something similar to the following figure in your browser:

Figure 3.33: Styled introduction section
Exercise 3.05: Product Cards
In this exercise, we will step through the process of writing the HTML and CSS for the product card component shown in the wireframe. The following figure shows the product card in more detail:

Figure 3.34: Product card
The steps to complete the exercise are as follows:
- First, let's create a file called product.html in VSCode using the following starter file:
<!DOCTYPE html>
<html>
<head>
<title>Exercise 3.05</title>
<style>
/* your CSS will go here */
</style>
</head>
<body>
<!-- your HTML will go here -->
</body>
</html>
- Next, let's use a div tag with a class name for the outer wrapper of the component. We will place this in between the opening and closing body tags as follows:
<body>
<div class="product-card">
</div>
</body>
- Now, let's add an image tag; we will add the image URL and some alt text:
<body>
<div class="product-card">
<img src="https://dummyimage.com/300x300/7EC0EE/000&text=Product+Image+1" alt="Product image 1" />
</div>
</body>
- We will then use h2 for the heading. Notice how we wrap the text in an anchor so that the user will be able to click on the card:
<body>
<div class="product-card">
<img src="https://dummyimage.com/300x300/7EC0EE/000&text=Product+Image+1" alt="Product image 1" />
<h2><a href="">Video title 1</a></h2>
</div>
</body>
- Now we will add the markup for the pricing information. Note that we will add a class name to allow us to style the information individually:
<body>
<div class="product-card">
<img src="https://dummyimage.com/300x300/7EC0EE/000&text=Product+Image+1" alt="Product image 1" />
<h2><a href="">Video title 1</a></h2>
<p class="original-price">RRP: £18.99</p>
<p class="current-price">Price you pay <span>£9.99</span></p>
<p class="saving">Your saving £9</p>
</div>
</body>
- Let's now take a look at what this looks like in the browser without any styling added:
Figure 3.35: Default product card
- Now, let's add some styling for the product card container in between the style tags as follows. Notice how we give the product card a black border and some padding:
<style>
.product-card {
display: inline-block;
border: 1px solid black;
padding: 15px;
}
</style>
- Then, we will add some styling for the individual elements of the product card. Starting with the image element, we will ensure the width of the image stretches to 100% by adding the following code:
.product-card img {
width: 100%;
}
We will add margin to the level 2 header using the following code:
.product-card h2 {
margin: 30px 0 15px;
}
The following code styles the links:
.product-card a {
color: black;
text-decoration: none;
}
With the help of the following code we will style the paragraph element:
.product-card p {
line-height: 1.5;
}
We will add the following code to style the original price, current price, and your savings as per our expected wireframe shown in Figure 3.34:
.original-price {
color: gray;
text-transform: uppercase;
}
.current-price span {
font-weight: bold;
text-decoration: underline;
}
.saving {
color: green;
}
If you now right-click on the filename in VSCode on the left-hand side of the screen and select open in default browser, you will see the product component in your browser.
You should now see something similar to the following figure in your browser:

Figure 3.36: Styled product cardExercise 3.06: Putting It All Together
Now that we have built the individual parts of the product page, we have the fun task of putting them all together to assemble a web page. We will be able to reuse the code we have written already and will just need to make minor tweaks to the CSS to get the page looking good. Our aim will be to produce a web page that resembles the wireframe as shown in the following figure:

Figure 3.37: Wireframe of the expected output
The steps to complete the exercise are as follows:
- First, let's create a file called product-page.html in VSCode. We will use the following HTML page template, noting we will use an inline style sheet to make things easier:
<!DOCTYPE html>
<html>
<head>
<title>Video product page</title>
<style type="text/css">
* {
margin: 0;
padding: 0;
}
body {
font-family: sans-serif;
margin: 0 auto;
width: 1200px;
}
header {
align-items: center;
display: flex;
margin-bottom: 25px;
}
nav {
margin-left: 30px;
}
.product-cards {
display: grid;
grid-template-columns: auto auto auto;
margin-bottom: 30px;
}
/* your styles will go here */
</style>
</head>
<body>
<header>
<img src="https://dummyimage.com/200x100/000/fff&text=Logo" alt="" />
<!-- navigation will go here -->
</header>
<section>
<!-- breadcrumb will go here -->
</section>
<!-- introduction section will go here -->
<section class="product-cards">
<!-- product cards will go here -->
</section>
</body>
</html>
- Now, let's add the navigation CSS, same as the code used in Exercise 3.02, Navigation, for the following HTML from the same exercise:
<nav>
<ul>
<li><a href="">Videos to rent</a></li>
<li><a href="">Videos to buy</a></li>
<li><a href="">Used DVDs</a></li>
<li><a href="">Offers</a></li>
</ul>
</nav>
- Then, we will add the styles for the breadcrumb component same as that of Exercise 3.03, Breadcrumb, for the following HTML from the same exercise:
<section>
<ol class="breadcrumb">
<li><a href="">Home</a></li>
<li><a href="">Used DVDs</a></li>
<li>Less than £10</li>
</ol>
</section>
- We will then add the introduction section's CSS same as that of Exercise 3.04, Page Heading and Introduction, for the following HTML from the same exercise:
<section class="intro">
<h1>Videos less than £10</h1>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. In bibendum non purus quis vestibulum. Pellentesque ultricies quam lacus, ut tristique sapien tristique et.</p>
</section>
- Finally, we will add the product card's CSS same as that of Exercise 3.05, Product Cards, for the following HTML from the same exercise:
<section class="product-cards">
<div class=»product-card»>
<img src="https://dummyimage.com/300x300/000/fff&text=Product+Image+1" alt="" />
<h2><a href="">Video title 1</a></h2>
<p class=»original-price»>RRP: £18.99</p>
<p class=»current-price»>Price you pay <span>£9.99</span></p>
<p class=»saving»>Your saving £9</p>
</div>
<-- Similar to the above product card 1 that is video title 1, the product cards for video titles 2, 3, and 4 can be copied from Exercise 3.05, Product Cards -->
</section>
If you now right-click on the filename in VSCode on the left-hand side of the screen and select open in default browser, you will see the web page.
You should now see something similar to the following figure in your browser:

Figure 3.38: Styled product page
Activity 3.01: Converting a Newspaper Article to a Web Page
Using a copy of a recent newspaper, choose a particular article and note down what HTML elements would be used if the paper were a web page. Now create a web page version of the newspaper article using semantic markup and CSS to recreate the layout as closely as possible:
- Get a copy of a newspaper article and annotate it with a pen to label the individual HTML elements.
- Create a file named home.html in VSCode. You can use the starter HTML from a previous exercise as a starting point.
- Start writing out the HTML for the news article.
- Now style the text and layout using CSS.
An example of how you could annotate a newspaper article to distinguish the different page elements can be seen in the following figure:

Figure 3.39: Sample annotated article
Note
You can find the complete solution in page 585.