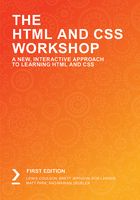
CSS Page Layouts
CSS provides us with a range of possibilities for laying out web pages. We will be looking into the three most common techniques for laying out web pages. These are as follows:
- float
- flex
- grid
Armed with this knowledge, combined with your knowledge of HTML structural tags, you will be able to code a range of web page layouts. The concepts learned in this part of the chapter will form the core of your frontend development skillset and you will use these techniques over and over throughout your career.
Video Store Product Page
In order to gain a solid understanding of how these three different approaches to layout work, we shall use a video store product listing page as a concrete example. We will work through solutions to the following design using the three most common layout techniques, one by one. For the examples that follow, we will only be concerned with the product section of the page:

Figure 2.15: Product page wireframe
Float-Based Layouts
The float-based CSS layout technique is the oldest of the three. Whilst CSS provides us with improved techniques for layout, the float-based layout is still used today. Having a firm grasp of how float-based layouts work in practice will set you up for more advanced styling segments in this book.
The float Property
The CSS float property, when applied to an element, will place the element to either the left or right of its containing element. Let's examine a few examples of the most common values for this property.
To float elements to the right, you would use the right value, as shown in the following code:
float: right;
Whereas, to float elements to the left, you would use the left value, as shown in the following code:
float: left;
The none value isn't used as frequently but, with the following code, it can be handy if you wish to override either the left or right values:
float: none;
The width Property
When we apply the float property to elements, we typically will also want to give the element an explicit width value as well. We can either give a value in pixels or percentages. The following code shows the input for width in pixels, that is, by writing px after the value:
width: 100px;
Whereas the following code shows the input for width as a percentage, that is, by entering the % symbol after the value:
width: 25%;
Clearing Floated Elements
As the name suggests, floated elements do, in fact, appear to float in relation to the other non-floated elements on the page. A common issue with floated elements inside a container is illustrated in the following figure:

Figure 2.16: Floating elements' illustration
Note
This solution of clearing floated elements has been used for simplicity.
There are many solutions to this issue, but by far the easiest solution is to apply the following CSS to the containing element:
section {
overflow: hidden;
}
With the preceding code added to the container, we will now have floated elements contained inside the wrapping element, as illustrated in the following figure:

Figure 2.17: Cleared floats' illustration
The following example code shows how you could achieve the preceding layout using float:
<!-- HTML -->
<section>
<div>product 1</div>
<div>product 2</div>
<div>product 3</div>
<div>product 4</div>
<div>product 5</div>
<div>product 6</div>
<div>product 7</div>
<div>product 8</div>
</section>
/* CSS */
section {
overflow: hidden;
}
div {
float: left;
width: 25%;
}
Flex-Based Layouts
The flex-based CSS layout technique is a new and improved alternative to the float-based approach. With flex, we have much more flexibility and can easily achieve complex layouts with very little code. With flex, we no longer have to worry about clearing floating elements. We will now look into some of the key properties and values in order to let us build the product page layout using flex.
The flex Container
When developing flex-based layouts, there are two key concepts you must first understand. The first is the flex container, which is the element that contains the child elements. To activate a flex layout, we must first apply the following code to the container or parent element that holds the individual items:
display: flex;
We also have to choose how we want the container to handle the layout of the child elements. By default, all child elements will fit into one row. If we want the child elements to show on multiple rows, then we need to add the following code:
flex-wrap: wrap;
The flex Items
Now that we know how to set the flex container up, we can turn to the child elements. The main issue of concern here is the need to specify the width of the child elements. To specify this, we need to add the following code:
flex-basis: 25%;
You can think of this as being equivalent to the width in our float-based example.
The following example code shows how you could achieve the product layout, as shown in Figure 2.15, using flex:
<!-- HTML -->
<section>
<div>product 1</div>
<div>product 2</div>
<div>product 3</div>
<div>product 4</div>
<div>product 5</div>
<div>product 6</div>
<div>product 7</div>
<div>product 8</div>
</section>
/* CSS */
section {
display: flex;
flex-wrap: wrap;
}
div {
flex-basis: 25%;
}
Grid-Based Layouts
The grid-based CSS layout technique is the newest of the three different approaches we will be exploring. This new approach was introduced in order to simplify the page layout and offer developers even more flexibility vis-à-vis the previous two techniques. We will now look into some of the key properties and values to enable us to build the product page layout using a grid-based approach.
The grid Container
When developing grid-based layouts, there are two key concepts you must first understand. The first is the grid container, which is the element that contains the child elements. To activate a grid layout, we must first apply the following code to the parent element:
display: grid;
Now that we have activated the container to use the grid-based layout, we need to specify the number, and sizes, of our columns in the grid. The following code would be used to have four equally spaced columns:
grid-template-columns: auto auto;
The grid Items
When we used float and flex layouts, we had to explicitly set the width of the child elements. With grid-based layouts, we no longer need to do this, at least for simple layouts.
We will now put our new-found knowledge into practice and build the product cards shown in Figure 2.15. We will use the grid layout technique since the product cards are actually within a grid layout, comprising four equally spaced columns.
Exercise 2.02: A grid-Based Layout
In this exercise, we will create our CSS page layout with the aim of producing a web page where six products are displayed as shown in the wireframe Figure 2.15.

Figure 2.18: Expected output for the grid based layout
Following are the steps to complete this exercise:
- Let's begin with the following HTML skeleton and create a file called grid.html in VSCode. Don't worry if you do not understand the CSS used here; you will soon enough:
<!DOCTYPE html>
<html>
<head>
<title>Grid based layout</title>
<style type="text/css">
div {
background: #659494;
color: white;
text-align: center;
margin: 15px;
padding: 100px;
}
</style>
</head>
<body>
</body>
</html>
- Next, we will add the product items using div tags, which are placed inside a section tag. We will just add a product with a number inside each item, so we know what product each represents:
<body>
<section>
<div>product 1</div>
<div>product 2</div>
<div>product 3</div>
<div>product 4</div>
<div>product 5</div>
<div>product 6</div>
<div>product 7</div>
<div>product 8</div>
</section>
</body>
- Now, let's add the following CSS in order to activate the grid-based layout. If you compare this to the other two techniques for laying out web pages, the code is very minimal:
section {
display: grid;
grid-template-columns: auto auto auto auto;
}
If you now right-click on the filename in VSCode on the left-hand side of the screen and select open in default browser you will see the web page in your browser repeat output for consistency.
If you now look at this page in your web browser, you should see a layout resembling the one shown in the screenshot.
We will now take a detour and look into some fundamental concepts of how CSS styles HTML elements.